Webページをクロールして最も頻繁に単語を取得するPythonプログラム
私たちのタスクは、Webページをクロールし、単語の頻度を数えることです。そして最終的に最も頻繁な単語を取得します。
まず、リクエストと美しいスープモジュールを使用し、これらのモジュールを使用してWebクローラーを作成し、Webページからデータを抽出してリストに保存します。
サンプルコード
import requests from bs4 import BeautifulSoup import operator from collections import Counter def my_start(url): my_wordlist = [] my_source_code = requests.get(url).text my_soup = BeautifulSoup(my_source_code, 'html.parser') for each_text in my_soup.findAll('div', {'class':'entry-content'}): content = each_text.text words = content.lower().split() for each_word in words: my_wordlist.append(each_word) clean_wordlist(my_wordlist) # Function removes any unwanted symbols def clean_wordlist(wordlist): clean_list =[] for word in wordlist: symbols = '!@#$%^&*()_-+={[}]|\;:"<>?/., ' for i in range (0, len(symbols)): word = word.replace(symbols[i], '') if len(word) > 0: clean_list.append(word) create_dictionary(clean_list) def create_dictionary(clean_list): word_count = {} for word in clean_list: if word in word_count: word_count[word] += 1 else: word_count[word] = 1 c = Counter(word_count) # returns the most occurring elements top = c.most_common(10) print(top) # Driver code if __name__ == '__main__': my_start("https://www.tutorialspoint.com/python3/python_overview.htm/")
出力
<中央>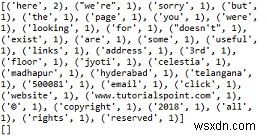
-
最も出現する文字とその数を見つけるPythonプログラム
この記事では、特定の問題ステートメントを解決するための解決策とアプローチについて学習します。 問題の説明 入力文字列が与えられた場合、最も出現する文字とその数を見つける必要があります。 アプローチ 文字列をキーとして、頻度を値として持つCounterメソッドを使用して辞書を作成します。 文字の最大出現回数、つまり値を見つけて、そのインデックスを取得します。 次に、以下の実装を見てみましょう- 例 from collections import Counter def find(input_): # dictionary &
-
Pythonのデータセットから最も頻繁に使用されるk個の単語を検索します
データセット内で最も頻繁に使用される10個の単語を検索する必要がある場合、Pythonはコレクションモジュールを使用してその単語を検索するのに役立ちます。 collectionsモジュールには、単語のリストを提供した後の単語の数を示すカウンタークラスがあります。また、most_commonメソッドを使用して、プログラム入力に必要な単語の数を調べます。 例 以下の例では、段落を取り、最初にsplit()を適用する単語のリストを作成します。次に、counter()を適用して、すべての単語の数を見つけます。最後に、most_common関数は、必要な頻度が最も高いそのような単語の数の適切な結果を提