AutoLayout を使用して iOS 用の Spotify クローンをプログラムで構築する方法
この投稿では、Spotify のホーム画面レイアウトを Swift でプログラムによって再作成しようとします。なぜプログラム的に?さまざまな方法で物事を構築する方法を知ることは常に良いことだと思います。プログラムで物事を行うためのコードを書くのが好きです。これらのスキルは、チームで作業している場合やバージョン管理を使用している場合に特に役立ちます。

これがSpotifyのモバイルアプリの実際のホーム画面。この種のレイアウトを実現するには、 UICollectionView
を使用します 、そして TabBarController
を使用するかもしれません タブ ナビゲーターを作成することもできます。
基本要件 :まず、Xcode +10 と swift +4 がインストールされていることを確認してください。
Xcode を使用して新しい Xcode プロジェクトを作成することから始めましょう:
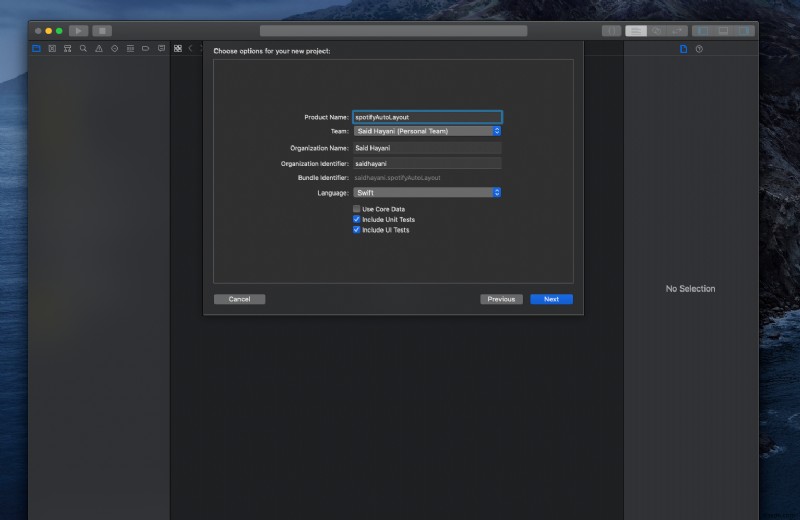
ViewController.swift
で最初に行う必要があるのは、 superClass を UICollectionViewController
に変更します UIViewController
の代わりに クラスは collectionView
に基づいているため .
//
// ViewController.swift
// spotifyAutoLayout
//
// Created by admin on 10/31/19.
// Copyright © 2019 Said Hayani. All rights reserved.
//
import UIKit
class ViewController: UICollectionViewController {
override func viewDidLoad() {
super.viewDidLoad()
collectionView.backgroundColor = .purple
// Do any additional setup after loading the view.
}
}
アプリを実行しようとすると、ビルドは失敗します。 AppDelegate.swift
にコードを追加する必要があります didFinishLaunchingWithOptions
内のファイル return
の前のこのコード部分を過ぎた関数 ステートメント:
let layout = UICollectionViewFlowLayout()
window = UIWindow()
window?.rootViewController = ViewController(collectionViewLayout: layout)
コードは次のようになります:
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
let layout = UICollectionViewFlowLayout()
window = UIWindow()
window?.rootViewController = ViewController(collectionViewLayout: layout)
return true
}
これで、アプリを実行して backgroundColor
を確認できるはずです purple
に変更 :
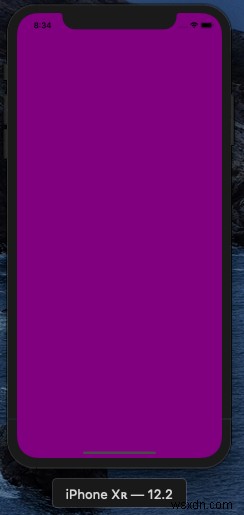
次のステップは、レイアウトを分散させ、セクション間でスペースを均等に分割することです。
CollectionView
のメソッドを定義しましょう .
手順:
- 一意の識別子を持つ再利用可能なセルを登録する
- セクション内の項目数を定義する
- 登録したセルを使用する
CollectionView
の一部を使用するには UICollectionViewDelegateFlowLayout
に常に準拠する必要があるメソッド スーパークラスとして、メソッドの autoComplete を取得します。それでは、CollectionViewCell の登録から始めましょう。
View.DidLoad()
内 collectionView.register()
と呼びます 再利用可能なセルを登録するメソッド:
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: cellId)
次に、collectionView
内にあるセルの数を定義します。 numberOfItemsInSection
を使用 .今のところ、5 つのアイテムにする必要があります:
override func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 5
}
次のステップは、cellForItemAt
を使用して再利用可能なセルを定義することです UICollectionViewCell
を返す必要があります cellId
という一意の ID を持っています .コードは次のようになります:
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: cellId, for: indexPath)
cell.backgroundColor = .red
return cell
}
完全なコードは次のようになります:
import UIKit
class ViewController: UICollectionViewController, UICollectionViewDelegateFlowLayout {
let cellId : String = "cellId"
override func viewDidLoad() {
super.viewDidLoad()
collectionView.backgroundColor = .purple
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: cellId)
}
override func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 5
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: cellId, for: indexPath)
cell.backgroundColor = .red
return cell
}
}
画面に赤い背景の 5 つのアイテムが表示されるはずです:
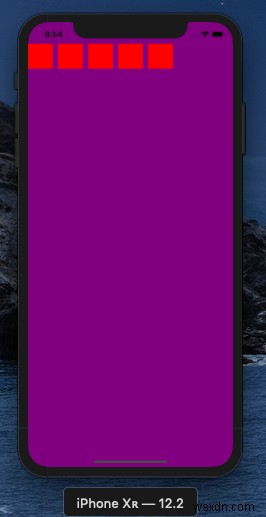
カスタムの幅と高さをセルに追加
次に、セルを正しい順序で配置し、width
を指定する必要があります。 と height
.各セルは width
を受け取ります 画面の width
.
sizeForItemAt
を持っていることは幸運です メソッドを使用して、セルにカスタムの width
を与えることができます と height
. CGSize
を返すメソッドです タイプ:
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
let width = view.frame.width
let height = CGFloat(200)
return CGSize(width: width, height: height)
}
そこで Cell
を作りました width
を取る view.frame.width
を使用して画面の カスタム height
は CGFloat
です タイプしてください。
シミュレータで以下の結果を確認できます:
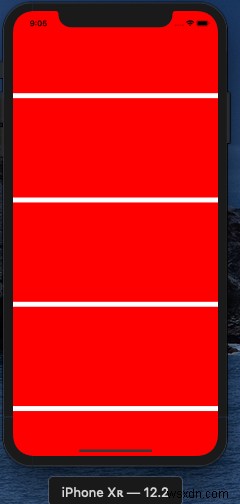
これまでのところ、すべてが良さそうです。今回は、再利用可能なカスタム セルを作成してみましょう。 CustomCell
という名前の新しい Swift ファイルを作成します :
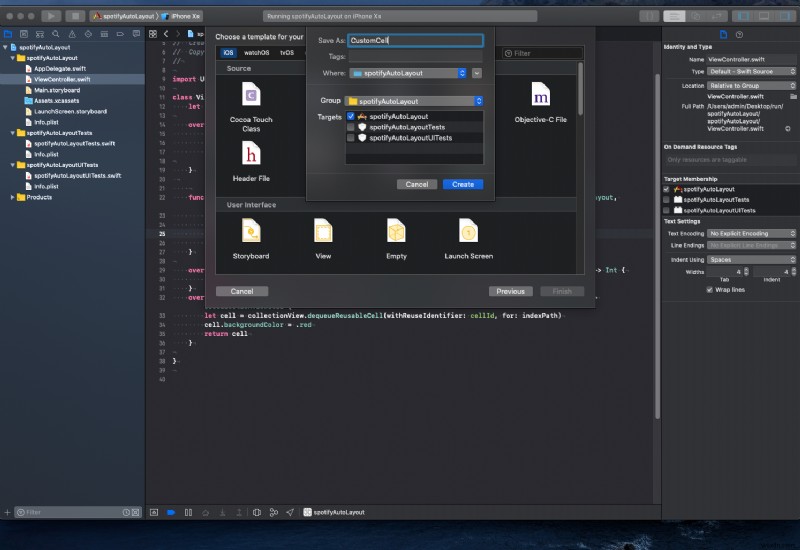
CustomCell.swift
以下のようになります:
import UIKit
class CustomCell: UICollectionViewCell {
override init(frame: CGRect) {
super.init(frame: frame)
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
次に、再利用可能なセル collectionView.register
をサポートするために 2 つのメソッドを変更する必要があります。 と cellForItemAt
.最初に register メソッドを変更しましょう。 UICollectionViewCell.self
を置き換えます CustomCell
で :
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: cellId)
次に cellForItemAt
をキャストする必要があります CustomCell
に準拠する 以下のように:
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: cellId, for: indexPath) as! CustomCell
アプリを実行しても、おそらく何の変化も見られないので、CustomCell に backgroundColor backgroundColor = .yellow
を指定します。 .行 cell.backgroundColor = .red
を削除することを忘れないでください cellForItemAt
で .背景色が黄色に変わったことがわかりますか?
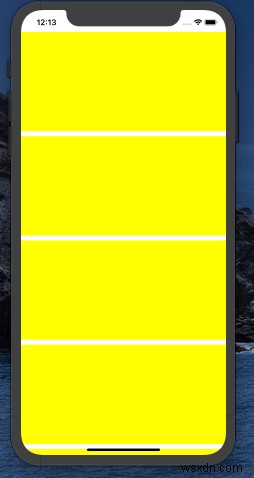
CutomCell
に塩を入れる時が来ました :D
Spotify のホーム画面を見ると、CustomCell
である各セクション この例では、セクション タイトル、サブセルが含まれており、横になっています:

セクション タイトルを追加
セルにタイトル ラベルを追加しましょう。 titleLabel
を作成します CutomCell
内の要素 クラス:
let titleLabel: UILabel = {
let lb = UILabel()
lb.text = "Section Title"
lb.font = UIFont.boldSystemFont(ofSize: 14)
lb.font = UIFont.boldSystemFont(ofSize: 14)
return lb
}()
次に、 init()
内のビューに要素を追加します ブロック:
addSubview(titleLabel)
アプリを実行しても何も変化が見られません。これは、要素にまだ制約を加えていないためです。それでは、いくつかの制約を追加しましょう – このプロパティ lb.translatesAutoresizingMaskIntoConstraints = false
を追加します へ titleLabel
要素に制約を適用できるようにする:
titleLabel
を追加した後 ビューに対して、制約を定義します:
addSubview(titleLabel)
titleLabel.topAnchor.constraint(equalTo: topAnchor, constant: 8).isActive = truetitleLabel.leftAnchor.constraint(equalTo: leftAnchor,constant: 8 ).isActive = true
必ず .isActive = true
を追加してください プロパティ – それなしでは、制約は機能しません!
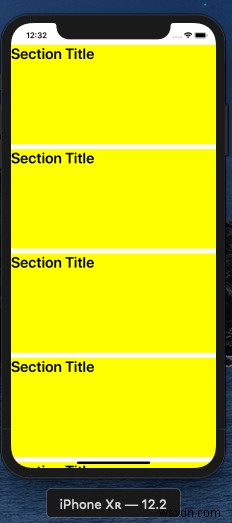
次の部分に進む前に、まず画面の背景色を黒に変更し、セルの黄色も削除しましょう:
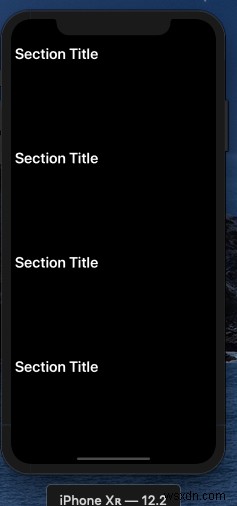
ここからは、各セルにサブセルを配置するという重要な部分です。それを達成するために、 CollectionView
を追加します CustomCell
内 .
CollectionView
を追加するには UICollectionViewCell
内 プロパティ UICollectionViewDelegate
を追加する必要があります 、 UICollectionViewDelegateFlowLayout
、および UICollectionViewDataSource
CustomCell
へのスーパークラスとして .
collectionView
を作成しましょう 単純なビューとしての要素:
let collectionView : UICollectionView = {
// init the layout
let layout = UICollectionViewFlowLayout()
// set the direction to be horizontal
layout.scrollDirection = .horizontal
// the instance of collectionView
let cv = UICollectionView(frame: .zero, collectionViewLayout: layout)
// Activate constaints
cv.translatesAutoresizingMaskIntoConstraints = false
return cv
}()
layout
を追加していることに注意してください collectionView
に viewController.swift
で初めて行ったように、初期化子のレイヤーとして .ここで FlowLayout
の方向も指定します .horizontal
になる .
collectionView
を追加しましょう 要素をサブビューとしてビューに追加します。
コードをもう少しきれいにするために、それを行う関数を作成します。
fileprivate func setupSubCells(){
// add collectionView to the view
addSubview(collectionView)
collectionView.dataSource = self
collectionView.delegate = self
// setup constrainst
// make it fit all the space of the CustomCell
collectionView.topAnchor.constraint(equalTo: titleLabel.bottomAnchor).isActive = true
collectionView.leftAnchor.constraint(equalTo: leftAnchor).isActive = true
collectionView.bottomAnchor.constraint(equalTo: bottomAnchor).isActive = true
collectionView.rightAnchor.constraint(equalTo: rightAnchor).isActive = true
}
デリゲートを self
に設定してください collectionView
の場合 および dataSource も:
collectionView.dataSource = self
collectionView.delegate = self
次に、init
内で関数を呼び出します ブロックします。
Xcode は UICollectionViewDelegate
に準拠していないため、アプリをビルドしようとするといくつかのエラーが表示されます。 と UICollectionViewDelegateFlowLayout
プロトコル。これを修正するには、最初にサブセルを再利用可能なセルとして登録する必要があります。
クラスの先頭に変数を作成し、cellId
という名前を付けます。 セル識別子が必要なときに使用できるようにします:
let cellId : String = "subCellID"
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: cellId)
エラーを解消するためのメソッドがさらに 2 つ不足しています:numberOfItemsInSection
セクション内のセル数と cellForItemAt
を定義する 再利用可能なセルを定義します。これらのメソッドは collectionView
に必要です 正常に動作するには:
// number of cells
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 4
}
// reusable Cell
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: cellId, for: indexPath)
cell.backgroundColor = .yellow
return cell
}
結果は次のようになります:
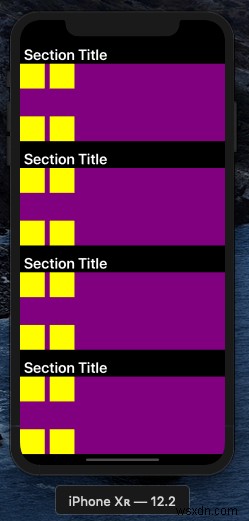
ご覧のとおり、collectionView
は背景として紫色で、サブセルは黄色です。
この記事を終える前にできる最後のことは make subCells
です セクションの高さと幅を持ちます。ここでも sizeForItemAt
を使用しています height
を定義する そして width
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
let width = frame.height
let height = frame.height
return CGSize(width: width, height: height)
}
そして、ここにいますか?:

良い!この記事は長くなりすぎないので、ここでやめておきます。 2 番目の部分を作成して、模擬写真を追加し、データを入力します。
完全なソース コード ?ここ
追加、質問、または訂正がある場合は、以下のコメントに投稿してください。または Twitter で連絡してください。
購読 このチュートリアルの第 2 部が公開されたときに通知を受けるために、私のメーリング リストに
-
iOS 13 でマウスを iPhone に接続する方法
最新の iOS バージョンのアップデート、つまり iOS 13 および iPadOS により、Bluetooth マウスを iPhone および iPad に接続できるようになりました。アクセシビリティ機能を使用して、マウスを iPhone に接続できるようになりました。この投稿では、マウスを iPhone に接続する方法について説明します。機能と進歩を改善するために、iOS デバイスでマウスを使用できます。これにより、外出先でドキュメントをすばやく処理できます。マウスを iPhone に接続して、インターネットをサーフィンできます。デスクトップと同じです。 iPhone でマウスを使用する方
-
iOS 16 搭載の iPhone でバッテリーのパーセンテージを表示する方法
5 年前、Apple は iPhone X を発売しました。これは、物議をかもした画面上部のノッチを備えたかなり斬新なデザインでした。 見た目は別として、ステータス バーのアイコンと情報に使用できるスペースが大幅に減少しました。そして犠牲者の 1 つは、Apple がバッテリーのパーセンテージを表示するオプションを削除したことです。 画面の右上から下にスワイプしてコントロール センターを表示することで、バッテリー残量の正確なパーセンテージを確認できます (今でも確認できます) が、これ (およびウィジェットを使用したその他の回避策) は、すべての人にとって満足できるものではありません.