React Native で動画を背景として使用する方法
この投稿では、backgroundVideo
を作成します。 React Nativeで。 React Native を使い始めたばかりの方は、私の記事 What you need to know to build mobile apps with React Native をチェックしてください。

バックグラウンド ビデオは、アプリの UI に素晴らしい効果を追加できます。ここで行うように、たとえば広告を表示したり、ユーザーにメッセージを送信したりする場合にも役立ちます。
いくつかの基本的な要件が必要になります。開始するには、react-native 環境のセットアップが必要です。つまり、あなたが持っていることを意味します:
- react-native-cli インストール済み
- Android SDK; Mac をお持ちの場合は必要ありません。Xcode だけです
はじめに
まず最初に、新しい React Native アプリをブートストラップしましょう。私の場合、react-native-cli を使用しています。ターミナルで以下を実行します:
react-native init myapp
これにより、React Native アプリを実行するためのすべての依存関係とパッケージがインストールされます。
次のステップは、シミュレーターでアプリを実行してインストールすることです。
iOS の場合:
react-native run-ios
これにより、iOS シミュレーターが開きます。
Android の場合:
react-native run-android
Android で問題が発生する場合があります。 Genymotion と Android エミュレーターを使用するか、このわかりやすいガイドを参照して環境をセットアップすることをお勧めします。
まず、Peleton アプリのホーム画面のクローンを作成します。 react-native-video
を使用しています ビデオストリーミング用、および styled-component
スタイリングに。したがって、それらをインストールする必要があります:
- 毛糸:
yarn add react-native-video styled-components
- NPM
npm -i react-native-video styled-components --save
次に、ネイティブ コードが含まれているため、react-native-video をリンクする必要があります — styled-components
の場合 それは必要ありません。単純に実行してください:
react-native link
他のことを心配する必要はありません。Video
に集中してください。 成分。まず、react-native-video からビデオをインポートして使用を開始します。
import import Video from "react-native-video";
<Video
source={require("./../assets/video1.mp4")}
style={styles.backgroundVideo}
muted={true}
repeat={true}
resizeMode={"cover"}
rate={1.0}
ignoreSilentSwitch={"obey"}
/>
分解してみましょう:
- ソース :ソース ビデオへのパス。代わりに URL を使用できます:
source={{uri:"https://youronlineVideo.mp4"}}
- スタイル: 動画に取り入れたいコスチューム スタイルと、背景動画を作成するための鍵
- resizeMode:この場合は
cover
です;contain or stretch
も試すことができます しかし、これでは私たちが望むものは得られません
その他の小道具はオプションです。
重要な部分に移りましょう:ビデオを背景位置に配置します。スタイルを定義しましょう。
// We use StyleSheet from react-native so don't forget to import it
//import {StyleSheet} from "react-native";
const { height } = Dimensions.get("window");
const styles = StyleSheet.create({
backgroundVideo: {
height: height,
position: "absolute",
top: 0,
left: 0,
alignItems: "stretch",
bottom: 0,
right: 0
}
});
ここで何をしましたか?
ビデオに position :absolute
を付けました ウィンドウに height
を指定します デバイスの。 Dimensions
を使用しました ビデオが全体のハイトを占めていることを確認するための React Native から — top:0, left:0,bottom:0,right:0
— ビデオがすべてのスペースを占有するように!
コード全体:
import React, { Component, Fragment } from "react";
import {
Text,
View,
StyleSheet,
Dimensions,
TouchableHighlight
} from "react-native";
import styled from "styled-components/native";
import Video from "react-native-video";
const { width, height } = Dimensions.get("window");
export default class BackgroundVideo extends Component {
render() {
return (
<View>
<Video
source={require("./../assets/video1.mp4")}
style={styles.backgroundVideo}
muted={true}
repeat={true}
resizeMode={"cover"}
rate={1.0}
ignoreSilentSwitch={"obey"}
/>
<Wrapper>
<Logo
source={require("./../assets/cadence-logo.png")}
width={50}
height={50}
resizeMode="contain"
/>
<Title>Join Live And on-demand classes</Title>
<TextDescription>
With world-class instructions right here, right now
</TextDescription>
<ButtonWrapper>
<Fragment>
<Button title="Create Account" />
<Button transparent title="Login" />
</Fragment>
</ButtonWrapper>
</Wrapper>
</View>
);
}
}
const styles = StyleSheet.create({
backgroundVideo: {
height: height,
position: "absolute",
top: 0,
left: 0,
alignItems: "stretch",
bottom: 0,
right: 0
}
});
// styled-component
export const Wrapper = styled.View`
justify-content: space-between;
padding: 20px;
align-items: center;
flex-direction: column;
`;
export const Logo = styled.Image`
max-width: 100px;
width: 100px;
height: 100px;
`;
export const TextDescription = styled.Text`
letter-spacing: 3;
color: #f4f4f4;
text-align: center;
text-transform: uppercase;
`;
export const ButtonWrapper = styled.View`
justify-content: center;
flex-direction: column;
align-items: center;
margin-top: 100px;
`;
export const Title = styled.Text`
color: #f4f4f4;
margin: 50% 0px 20px;
font-size: 30;
text-align: center;
font-weight: bold;
text-transform: uppercase;
letter-spacing: 3;
`;
const StyledButton = styled.TouchableHighlight`
width:250px;
background-color:${props => (props.transparent ? "transparent" : "#f3f8ff")};
padding:15px;
border:${props => (props.transparent ? "1px solid #f3f8ff " : 0)}
justify-content:center;
margin-bottom:20px;
border-radius:24px
`;
StyledTitle = styled.Text`
text-transform: uppercase;
text-align: center;
font-weight: bold;
letter-spacing: 3;
color: ${props => (props.transparent ? "#f3f8ff " : "#666")};
`;
export const Button = ({ onPress, color, ...props }) => {
return (
<StyledButton {...props}>
<StyledTitle {...props}>{props.title}</StyledTitle>
</StyledButton>
);
};

また、次の操作を行うことで、このコンポーネントを再利用可能にすることができます:
<View>
<Video
source={require("./../assets/video1.mp4")}
style={styles.backgroundVideo}
muted={true}
repeat={true}
resizeMode={"cover"}
rate={1.0}
ignoreSilentSwitch={"obey"}
/>
{this.props.children}
</View>
また、他のコンポーネントと一緒に使用できます:
それだけです。読んでくれてありがとう!
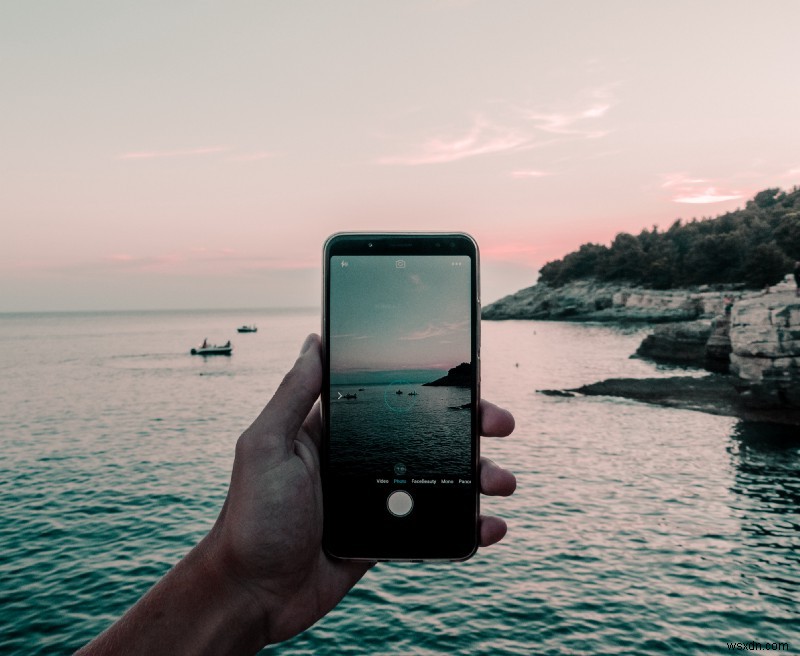
React Native の詳細:
- React Native でモバイル アプリの構築を開始するために知っておくべきこと
- React Native でのスタイリング
その他の投稿:
- JavaScript ES6、書くのを減らす — もっとやる
- Vue.js でルーティングを使用してユーザー エクスペリエンスを向上させる方法
- JavaScript で HTTP リクエストを行う最も一般的な方法は次のとおりです
Twitter で私を見つけることができますか?
私のメーリング リストに登録して、今後の記事に注目してください。
-
Windows 10 または 11 でビデオ壁紙を使用する方法
しばらくの間 Windows オペレーティング システムに手を出している場合は、デスクトップまたは背景画像を少なくとも 1、2 回微調整したに違いありません。そして、なぜですか? Web には、あらゆる種類の魅力的な画像があふれています。自然や宇宙から抽象や科学まで、Unsplash、Pexels などの無料の写真 Web サイトでは、さまざまな写真から選択できます。 ただし、画像が優れているのと同様に、Windows には別のオプション、つまりビデオの壁紙もあります。この記事では、ビデオの壁紙を Windows PC のデスクトップの背景として設定する方法について説明します。 Window
-
iPhone 8 の新しいビデオ形式の使い方
最近発売された iPhone 8 と iPhone 8 Plus には、初めて搭載された優れた機能があります。最も注目すべき機能には、1080P Slo-mo ビデオで 60 フレーム/秒 (FPS) および 240 FPS の 4K ビデオを撮影できる 2 つのビデオ形式があります。 以前の iPhone モデルでは、ユーザーは FPS が低い Ultra HD モードでしか撮影できませんでした。しかし、技術の進歩によりすべてが変わり、Ultra HD でより多くのフレームをキャプチャできるようになりました。 2 つのビデオ形式は高品質のビデオをキャプチャしますが、以前の形式と比較して